How to write WebMatrix Add-Ins
The main functionality of Add-Ins in Matrix is exposed in the Microsoft.Matrix.Core.Plugins
namespace.
There are two types of Add-Ins in Matrix (at least that I know):
-
Global Add-Ins: These are Add-Ins that are always enabled and that can be
executed without the requirement of having a document (window) opened in Matrix.
These are essentially classes that are derived from Microsoft.Matrix.Code.Plugins.AddIn.
-
Document Add-Ins: These are Add-Ins that are only enabled in the context
of a document, only when the user has a document opened in Matrix. These are classes
that are derived from Microsoft.Matrix.Code.Plugins.DocumentAddIn.
Creating the simplest Add-In
Using VS.NET
In VS.NET create a new project of type Library Application:
-
Select the option New Project from the File Menu
-
Select the language of your choice (we will use C#)
-
Select Class Library In the templates list
-
Specify the name and the location where you want files. In this case we will use
Samples.AddIns as the name.
-
Click Ok.
|
|
Add a reference to System.Windows.Forms and Microsoft.Matrix.dll located
at: <Program Files>\Microsoft ASP.NET Web Matrix\v<version>\
for example, in my machine is located at:
C:\Program Files\Microsoft ASP.NET Web Matrix\v0.6.812\
-
Select the option Add reference from the Project Menu
-
Click Browse button to find Microsoft.Matrix.dll, and click Ok.
-
From the Component Name List select System.Windows.Forms.dll and click Select.
-
Click Ok to close the dialog.
|
|
Modify the code of the class1.cs file to look as follows:
|
using System;
using System.Windows.Forms;
using Microsoft.Matrix.Core.Plugins;
namespace Samples.Addins {
[Plugin("My First AddIn",
"This is my first addin",
"carlosag", "me@carlosag.net")]
public class FirstAddIn
: AddIn {
protected override void Run() {
MessageBox.Show("Hello
World");
}
}
}
The code above defines a class that derives from Microsoft.Matrix.Core.Plugins.AddIn,
that will create a global add-in for Matrix. In this case the only thing we are
doing is override the Run method that gets invoked whenever the add-in needs to
do its thing. Usually you will not override this method, and only override the CreateAddInForm
that is called from the default implementation of Run, but we will see that later.
This add-in will only display a MessageBox when the addin is executed.
Now you should be able to compile it using the option “Build Solution” from the
“Build” Menu.
If you are using only the .NET Framework you can compile it using the following
command line:
csc.exe /t:library /out:Samples.AddIns.dll "/r:d:\Program Files\Microsoft
ASP.NET Web Matrix\v0.6.812\Microsoft.Matrix.dll" Class1.cs
Now that we have the Dll we just need to register it in Matrix.
Registering the AddIn in Matrix:
Launch Matrix from “StartàProgramsàMicrosoft
ASP.NET Web MatrixàASP.NET Web Matrix”
|

|
Select the option “Organize Add-ins…” from the Tools menu
|
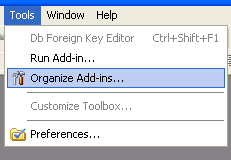
|
Click the “Add Local” button
|
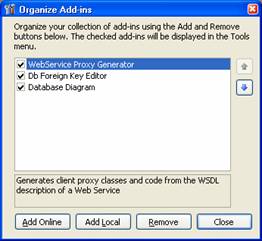
|
In the Select Components dialog, click the “Browse…” button and search for the Samples.AddIns.dll.
Click Ok. Click Ok.
|
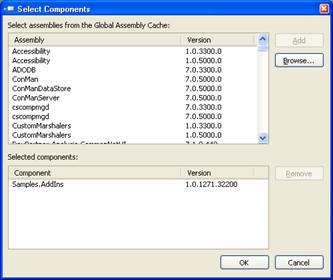
|
Back in the ”Organize Add-ins”, you will see the add-in that was just registered,
mark the checkbox to make this add-in available from the Tools menu.
|
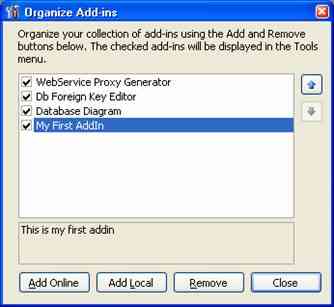
|
Click Close.
|
|
By this time, you should have a new option inside the Tools menu that shows the
Name of your AddIn. Select the option :”My First AddIn”, and your code will be run.
|
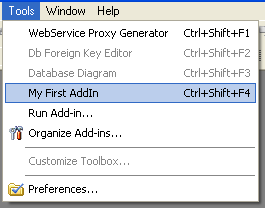
|